Understanding Docker Images and Containers 🛠️
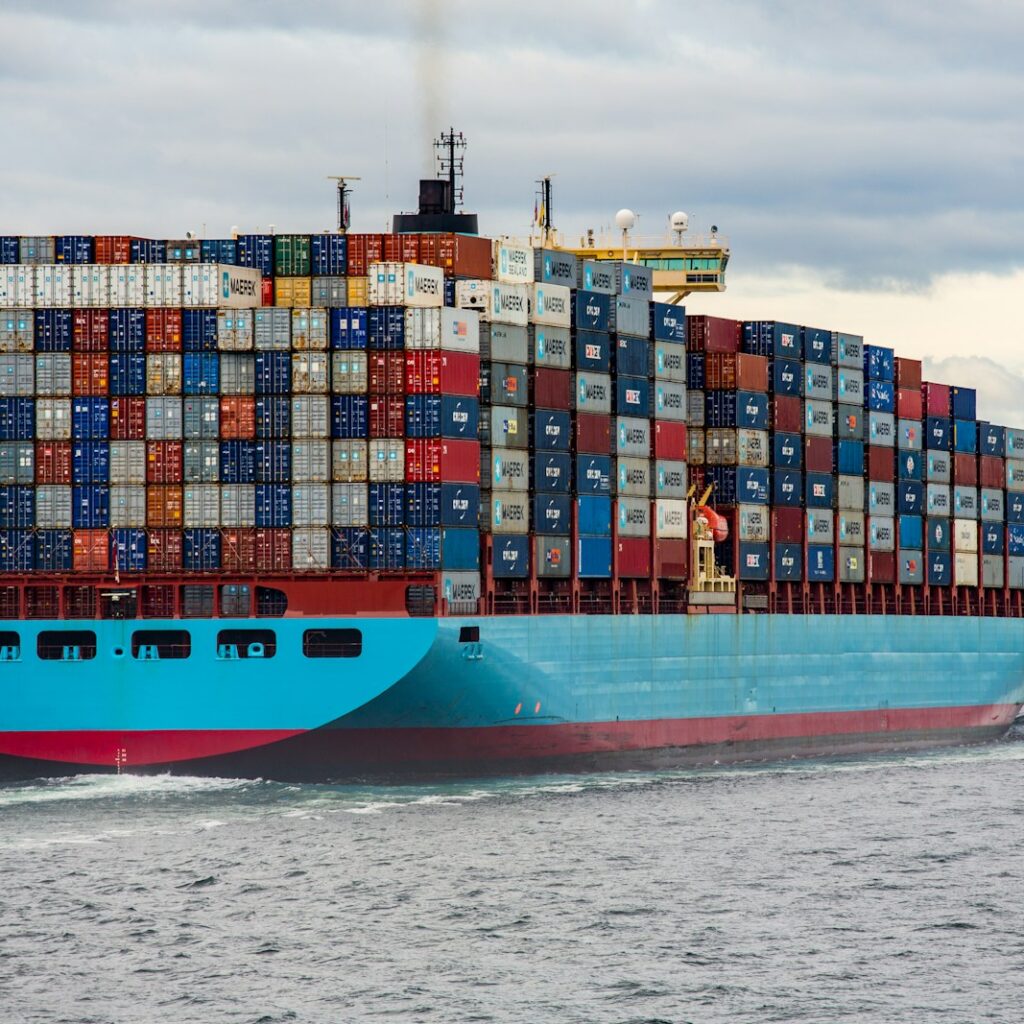
Now that you have Docker installed and ready to go, it’s time to dive deeper into two of the most fundamental concepts in Docker: Images and Containers. These are the building blocks that make Docker such a powerful tool for modern application development and deployment.
What are Docker Images?
Docker Images are the blueprints for your containers. Think of an image as a snapshot of everything your application needs to run—this includes the application code, runtime, libraries, environment variables, and dependencies. Images are immutable, meaning once they’re created, they don’t change. This immutability ensures that every time you run a container from the same image, it behaves exactly the same way, regardless of where it’s executed.
Key characteristics of Docker Images:
- Layered Structure: Docker images are built in layers. Each layer represents a step in the building process, such as adding files, installing software, or setting up environment variables. These layers make Docker images efficient because they allow for reusability and reduce redundancy. If multiple images share common layers, Docker only needs to store those layers once, saving disk space and speeding up deployments.
- Base Images: A Docker image can be built from a base image, such as an official OS image (like Ubuntu or Alpine) or a specific runtime environment (like Python or Node.js). From this base, additional layers are added to create a fully functional application image.
- Dockerfile: The blueprint for creating a Docker image is defined in a Dockerfile. This file contains a series of instructions that tell Docker how to build the image, such as which base image to use, which files to copy, and which commands to run.
What are Docker Containers?
While Docker Images are the blueprints, Docker Containers are the running instances of these images. A container is essentially a lightweight, standalone, and executable package of software that includes everything needed to run it: code, runtime, system tools, libraries, and settings.
Key characteristics of Docker Containers:
- Isolation: Containers provide process and filesystem isolation. This means that each container runs independently, with its own set of processes and filesystem, separate from the host machine and other containers. This isolation makes containers ideal for running multiple applications on the same host without conflicts.
- Portability: Because containers encapsulate everything the application needs to run, they can be moved across different environments—development, testing, production—without worrying about compatibility issues. If it works on your machine, it will work on any machine that runs Docker.
- Ephemeral Nature: Containers are designed to be ephemeral, meaning they can be stopped, started, and even destroyed without impacting the underlying image. This makes it easy to spin up new containers as needed, scale applications, and manage resources efficiently.
Building and Running Containers
Let’s walk through the process of building a Docker image and running a container:
1. Building a Docker Image
To create a Docker image, you’ll typically start by writing a Dockerfile. Here’s an example Dockerfile for a simple Node.js application:
# Use an official Node.js runtime as a base image
FROM node:14
# Set the working directory inside the container
WORKDIR /usr/src/app
# Copy the package.json file and install dependencies
COPY package*.json ./
RUN npm install
# Copy the rest of the application code
COPY . .
# Expose the application port
EXPOSE 8080
# Define the command to run the application
CMD ["node", "app.js"]
With this Dockerfile in place, you can build the image using the following command:
docker build -t my-node-app .
Here, -t
tags the image with a name (my-node-app
), and .
specifies the current directory as the build context.
2. Running a Docker Container
Once you have your image, you can create and run a container from it:
docker run -d -p 8080:8080 my-node-app
This command does the following:
- -d: Runs the container in detached mode (in the background).
- -p 8080:8080: Maps port 8080 on your local machine to port 8080 inside the container.
- my-node-app: The name of the image you want to run.
After running this command, your Node.js application will be running inside a container, accessible via http://localhost:8080
.
Managing Docker Containers
Here are some essential commands for managing Docker containers:
docker ps
: Lists all running containers.docker ps -a
: Lists all containers, including stopped ones.docker stop [container_id]
: Stops a running container.docker start [container_id]
: Starts a stopped container.docker rm [container_id]
: Removes a stopped container.docker logs [container_id]
: View the logs of a running container.
Containers vs. Images: The Relationship
To sum it up:
- Docker Images are like blueprints or templates. They define what’s inside a container but are not active themselves.
- Docker Containers are the live, running instances created from Docker Images. You can think of containers as “active blueprints” that execute your code in a consistent and isolated environment.
Next Steps: Delving Deeper into Docker Commands and Networking
With a solid understanding of Docker images and containers, you’re now ready to explore more advanced Docker commands and how networking works in Docker. These topics will enable you to manage and scale your applications more effectively.
Let’s continue building your Docker expertise in the next lesson! 🚀
This lesson is part of the FREE full course